To print an exception in Python, you can either just call print(e)
directly on the exception object, or use traceback.print_exc()
to print the entire stack trace. You’ll have to import the traceback
module to use the second option, though.
How to print just the exception message
Printing exceptions is easy in Python. Since they all (should) implement the __repr__
“dunder” method, calling print()
on them will just print a user-friendly message, usually whatever the throwing code put as the error when they raised it.
Here’s an example:
try:
print("Hello world")
raise Exception("This is a problem!")
except Exception as e:
print(e)
PythonWe print out “Hello world” and then immediately raise an exception with “This is a problem!” as the message.
Here’s the output:
Hello world
This is a problem!
As you can see, all this prints out is the message we gave to Exception
when we instantiated it.
But, this isn’t so great for debugging since we don’t have a stack trace. So, how do we get that?
How to print the stack trace along with the exception
If you want to see the stack trace, you’ll have to import the traceback
module and use the print_exc()
function within it like this:
import traceback
try:
print("Hello world")
raise Exception("This is a problem!")
except Exception as e:
traceback.print_exc()
PythonYou might have noticed we don’t pass in the e
instance of the Exception
after we catch it; that’s because the traceback.print_exc()
function automagically knows what exception we’re dealing with.
Here’s the output:
Hello world
Traceback (most recent call last):
File "/home/user/main.py", line 5, in <module>
raise Exception("This is a problem!")
Exception: This is a problem!
And that’s all there is to printing a stack trace yourself rather than letting an exception go unhandled.
Conclusion
In Python, printing an exception is as easy as catching it (make sure you’re catching the right type!) and then calling print
on it. However, you might also want the stack trace. To do that, you simply import the traceback
module and call traceback.print_exc()
from within an except
block.
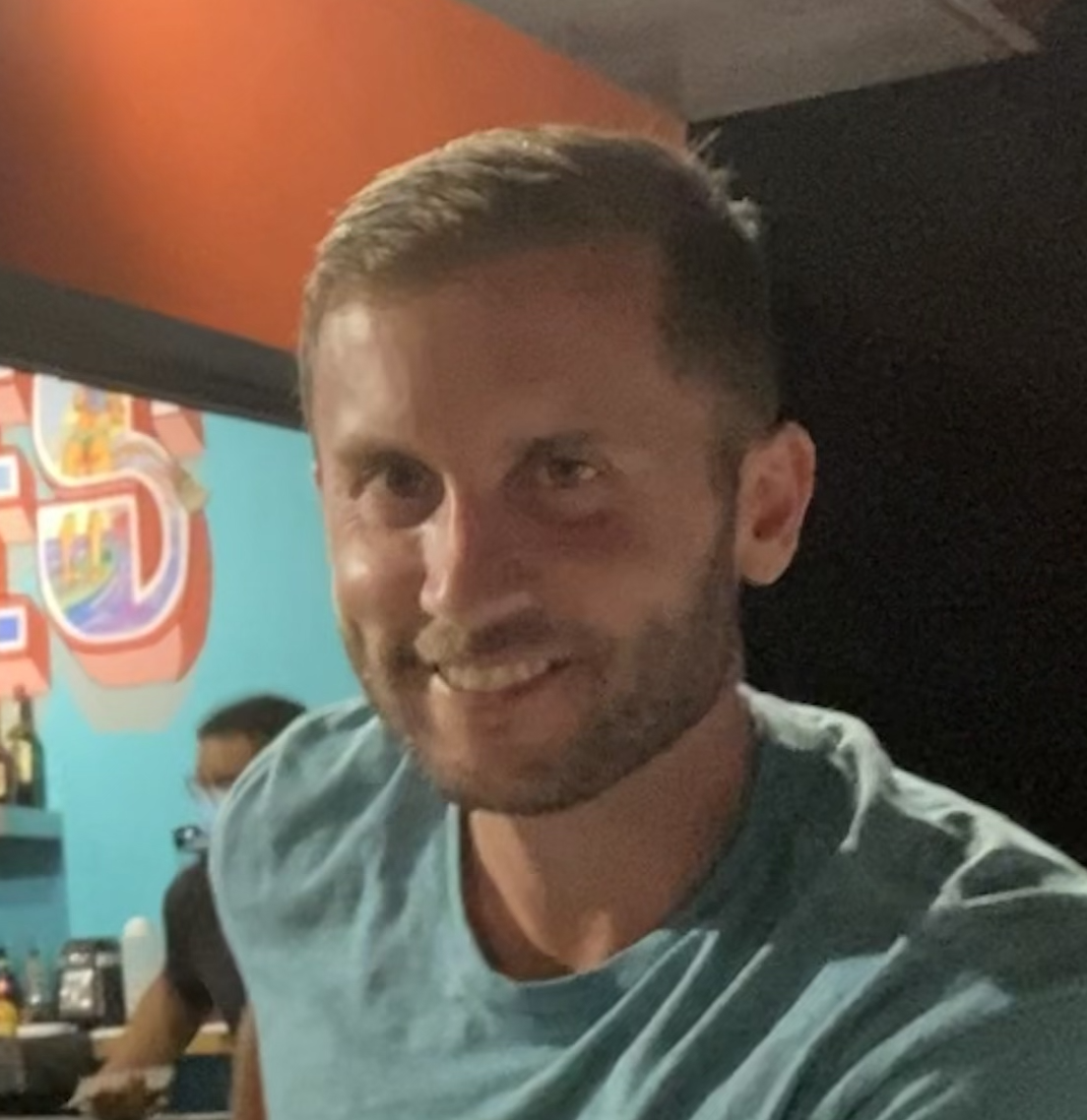
John is a professional software engineer who has been solving problems with code for 15+ years. He has experience with full stack web development, container orchestration, mobile development, DevOps, Windows and Linux kernel development, cybersecurity, and reverse engineering. In his spare time, he’s researching the potential business applications of AI.