Returning a list in Python is as simple as using the return statement from within a function, followed by an object of type list
. For example: return [1, 2, 3]
. This will return a list containing elements 1
, 2
, and 3
.
Table of Contents
Return a list directly
Returning a list in Python can be done by simply returning the definition of a list directly, as you can see below:
def foo():
return ["this", "is", "a", "list"]
print(foo())
PythonHere, we’ve created a function called foo
that just returns a list that is defined in-line with the return
statement. If we run this, we’ll get the following output:
['this', 'is', 'a', 'list']
Return a list by variable name
We can also return a variable that refers to a list in Python. This is done in basically the same way as the previous example, except we assign the list definition to a variable first before returning it. Obviously, this is just an example to show how the list can be returned, so there’s no benefit to assigning the list to a variable before returning it in the code below.
def foo():
bar = ["this", "is", "a", "list"]
return bar
print(foo())
PythonAs you can see, we assigned the same list definition as the first example to a variable named bar
and then returned it. Below is the output you’d get:
['this', 'is', 'a', 'list']
As expected, this is the same as just returning the list directly.
Return a list with list comprehension
Another way to return a list from a function in Python is to use list comprehension. This is a way to give Python a sort of recipe for a list rather than explicitly defining each element individually. It’s a very useful technique that can make your code a whole lot more readable than, say, building a list with a for
loop, for example.
Here’s how you’d do it:
def foo():
return [f"test {number}" for number in range(10)]
print(foo())
PythonWhat our list comprehension above is doing is telling Python to make a range
of numbers (using the built in range()
function), then take each one and put it into a string with the format "test {number}"
, where {number}
will be replaced with the current number from the range we’re working with.
Here’s the result:
['test 0', 'test 1', 'test 2', 'test 3', 'test 4', 'test 5', 'test 6', 'test 7', 'test 8', 'test 9']
As you can see the range()
function gives you by default a range starting with 0 and ending with the number you passed to it, minus one. Our list comprehension worked as expected.
Conclusion
Returning a list in Python is very simple – you can just do it. There’s no special way to return a list. It’s just an object like everything else and can be passed around as such.
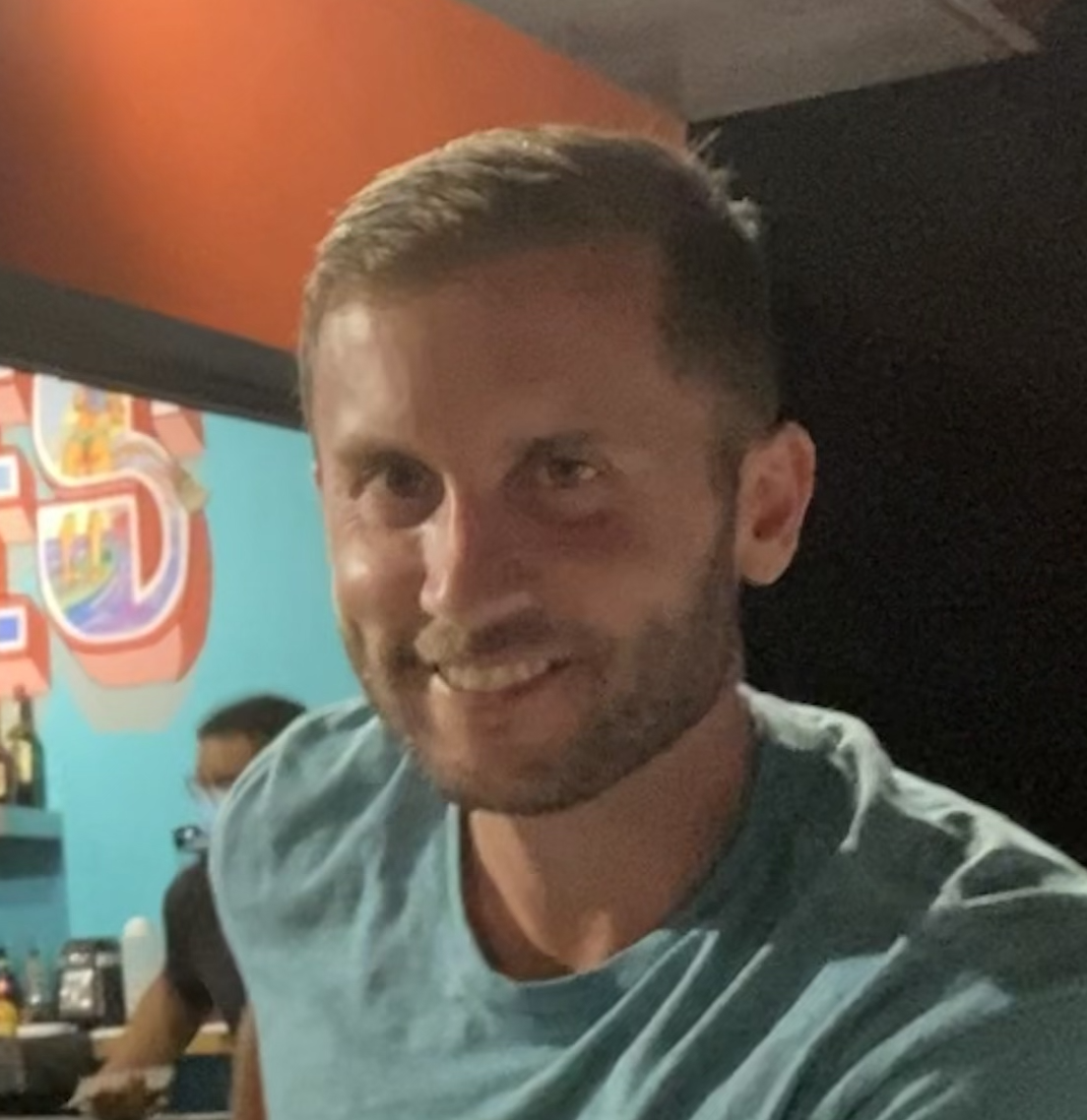
John is a professional software engineer who has been solving problems with code for 15+ years. He has experience with full stack web development, container orchestration, mobile development, DevOps, Windows and Linux kernel development, cybersecurity, and reverse engineering. In his spare time, he’s researching the potential business applications of AI.