The recommended way to remove http or https from an URL in Javascript is to use the built-in URL object and access the various parts of the URL that way. Alternatively, you can use the String.replace()
function with a regular expression and replace it with an empty string.
Table of Contents
Use the built-in URL object (recommended)
The URL class is supported in every major browser these days and is recommended for parsing URLs as well as accessing their various components. I think this is a lot better than trying to use a regular expression since there’s less likelihood you’ll forget an obscure edge case. Also, since it’s part of Javascript, it’s a very well-tested piece of code, unlike a regular expression you just threw together (no offense).
Get just the host
If you just want to get the host from the URL, do the following:
const raw_url = "https://www.example.com";
const url = new URL(raw_url);
console.log(url.host)
JavaScriptIn the above code, we use https://www.example.com
as our target URL, and assign it to the raw_url
variable. Then, we construct a URL
object out of it and assign that to the url
variable. Finally, we print out just the host
property of that URL
object, which gives us the following output:
www.example.com
Get the host and path
If the URL has a path portion to it that you also want, you can append the pathname
property of the URL object to the host
property like this:
const raw_url = "https://www.example.com/foo/bar";
const url = new URL(raw_url);
console.log(url.host + url.pathname)
JavaScriptWhich will give you the following:
www.example.com/foo/bar
Use a regular expression and the String.replace() function
If you’re dead set on using a regex instead of doing it the easy, well-tested, and robust way… here’s how you do that:
const raw_url = "https://www.example.com/foo/bar";
console.log(raw_url.replace(/https?:\/\//, ""))
JavaScriptUsing the same URL from the previous example, we make a regular expression that matches “http://” or “https://” (note the question mark after the “s”), and replace it with an empty string. That gives us the same result:
www.example.com/foo/bar
This will not match any random URI such as sftp://whatever
. This is only going to work for http
or https
URLs, which is another reason I highly recommend you just use the built-in URL class.
Conclusion
To remove the protocol portion (http:// or https:// usually) from a URL in Javascript, you should use the built in URL
class. If you’re confident you aren’t going to screw it up, you can use the String.replace
method with a regex matching the protocol and replace it with an empty string.
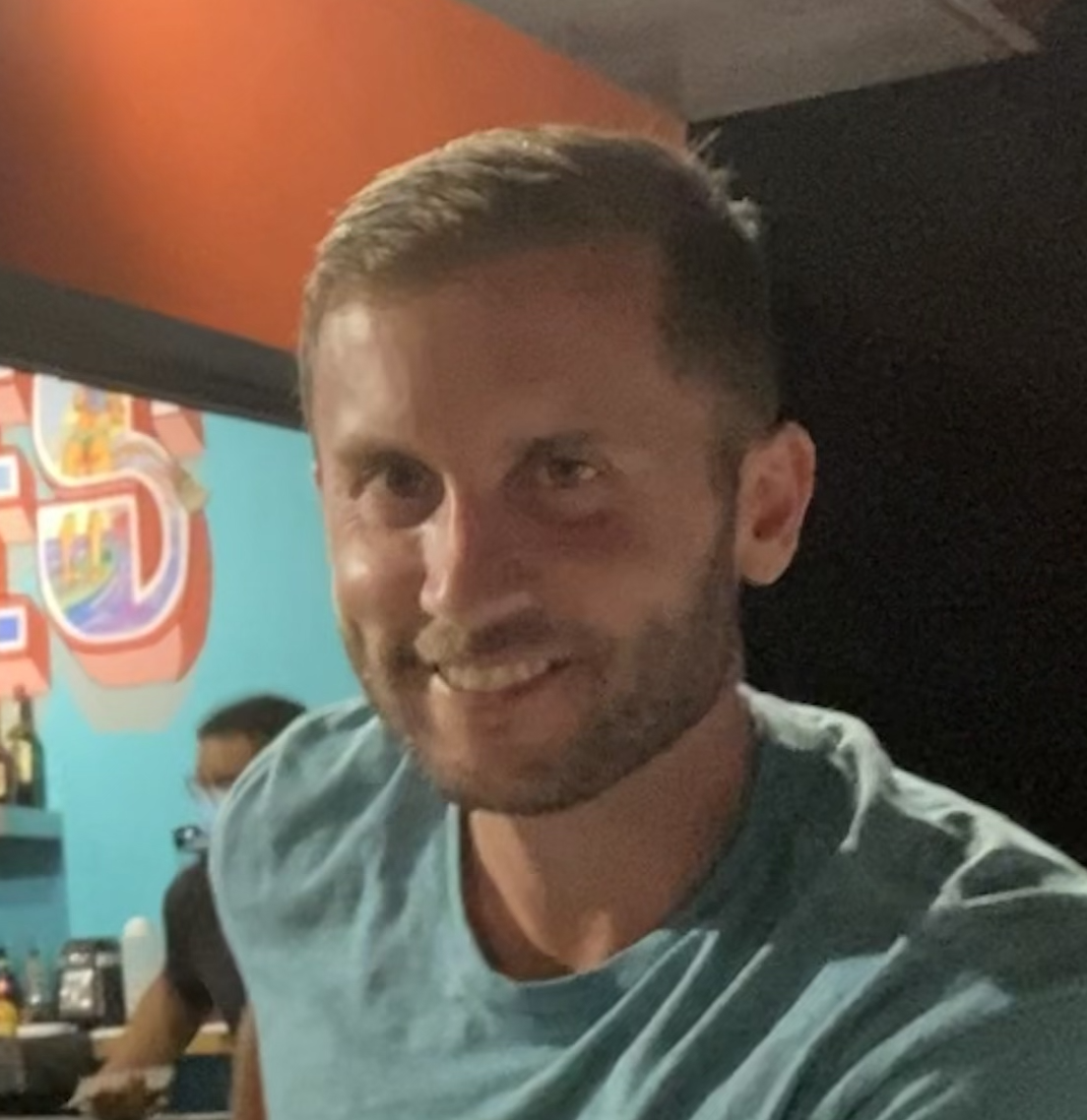
John is a professional software engineer who has been solving problems with code for 15+ years. He has experience with full stack web development, container orchestration, mobile development, DevOps, Windows and Linux kernel development, cybersecurity, and reverse engineering. In his spare time, he’s researching the potential business applications of AI.