In Python, semicolons (;) can be used to join two separate lines on a single line, and for
loops can have the body of the loop on the same line after the colon (:). Alternatively, you can use list comprehension to put a for
loop on a single line.
Table of Contents
Simply put it on one line
In Python, it’s correct syntax (though not personally recommended) to put the body of a for
loop on the same line as the for
statement itself, after the colon:
mylist = ["a", "b", "c"]
for item in mylist: print(item)
PythonAnd the output:
a
b
c
So, that works. But what if there’s more than just a print
statement in the for
loop?
What if the loop body is more than one line?
It’s also correct syntax in Python to put a semicolon between statements in order to put them on the same line. Again, I don’t recommend this in most cases, because it seriously degrades the readability of your code. But, if you really want to, here’s how you do that:
mylist = ["a", "b", "c"]
for item in mylist: print(item); print(f"Hello, {item}")
PythonHere we’re not only doing the plain print
statement on each list item, we’re also printing a simple format string on each item as well.
Here’s the output:
a
Hello, a
b
Hello, b
c
Hello, c
In fact, you can chain together as many Python statements as you want on one line, separated by a semicolon. Anyone reading your code will probably not appreciate this, though.
Use list comprehension
A more acceptable and “Pythonic” way to put a for
loop on one line is something called list comprehension. This is a way to create a list using a for
loop in a much more concise way than was required in ancient versions of Python.
Here’s how we used to have to do it back in the day:
mylist = []
for i in range(10):
mylist.append(i)
print(mylist)
PythonThe above code creates a list of numbers 0-9. Here’s the output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Kind of a pain, isn’t it?
With list comprehension, though, we can do this all on one line:
mylist = [i for i in range(10)]
print(mylist)
PythonWhich gives us the same output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Conclusion
Putting a for
loop all on one line is easy in Python; just put the code you would normally put beneath the for
loop on the same line after the colon. If you have more than one statement you need executed for each pass through the loop, separate them with semicolons. Alternatively, if the purpose of your loop is to create a list, you can use list comprehension to build that list on one line.
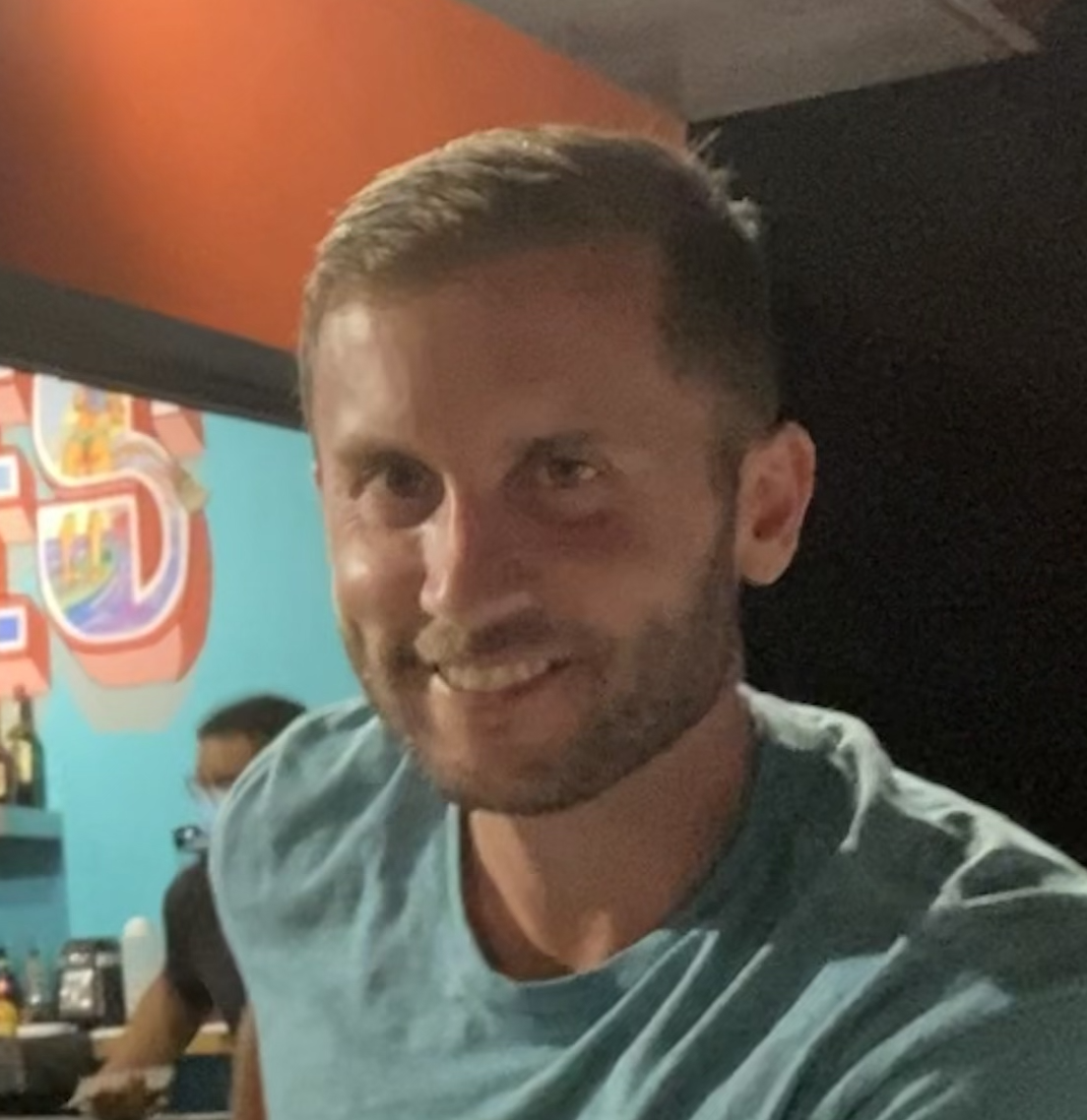
John is a professional software engineer who has been solving problems with code for 15+ years. He has experience with full stack web development, container orchestration, mobile development, DevOps, Windows and Linux kernel development, cybersecurity, and reverse engineering. In his spare time, he’s researching the potential business applications of AI.