This error means you tried to call .get
on a string. The str
type in Python doesn’t have that method. You probably meant to call .get
on a dict
instead. To fix this, make sure the variable you’re calling .get
on is a dict
and not a string.
The dict
type in Python has a convenient method called .get
that allows you to get items from it without throwing a stack trace if that item isn’t there. Not all types in Python have this method, and if you call it on a str
type, you’ll get this error message. Let’s take a look at how to generate this error and then how to solve it.
Table of Contents
Problem: the variable you’re calling get on is a str and not a dict
Below is super simple, contrived example. Your situation is probably more complicated, but not impossible to figure out:
my_string = "Hello world!"
my_string.get("foo")
PythonRunning this code will cause the following error message:
Traceback (most recent call last):
File "/home/user/main.py", line 2, in <module>
my_string.get("foo")
AttributeError: 'str' object has no attribute 'get'
Like it says, str
doesn’t have a method called get
.
Solution: check the type of the variable before calling get
It’s almost certain you thought this variable was a dict
(I hope), so one way to solve this is to do a type check before trying to call that method on it:
my_string = "Hello world!"
def do_something_with_a_dict(maybe_a_dict):
if type(maybe_a_dict) is dict:
print(maybe_a_dict.get("foo"))
else:
print("Error! That's not a dict!")
do_something_with_a_dict(my_string)
# -> Error! That's not a dict!
PythonThis is one easy way to make sure this error never happens. By using Python’s type()
function (built-in), you’ll be able to tell what the variable is that you’re working with before you try to do something with it you’re not supposed to.
In all likelihood though, this problem is because of some logic error in your programming. Take a look through your code and make sure the variables pointed to by your stack trace are what you think they are.
Conclusion
The Python error “AttributeError: ‘str’ object has no attribute ‘get'” simply means you tried to call .get()
on a string object. Those don’t have that method. dict
s do, however, and it’s probable that you were thinking the variable you were working with was a dict
. So, take a look through your code, and make sure that’s the case. If all else fails, do a type check with the built-in type()
function.
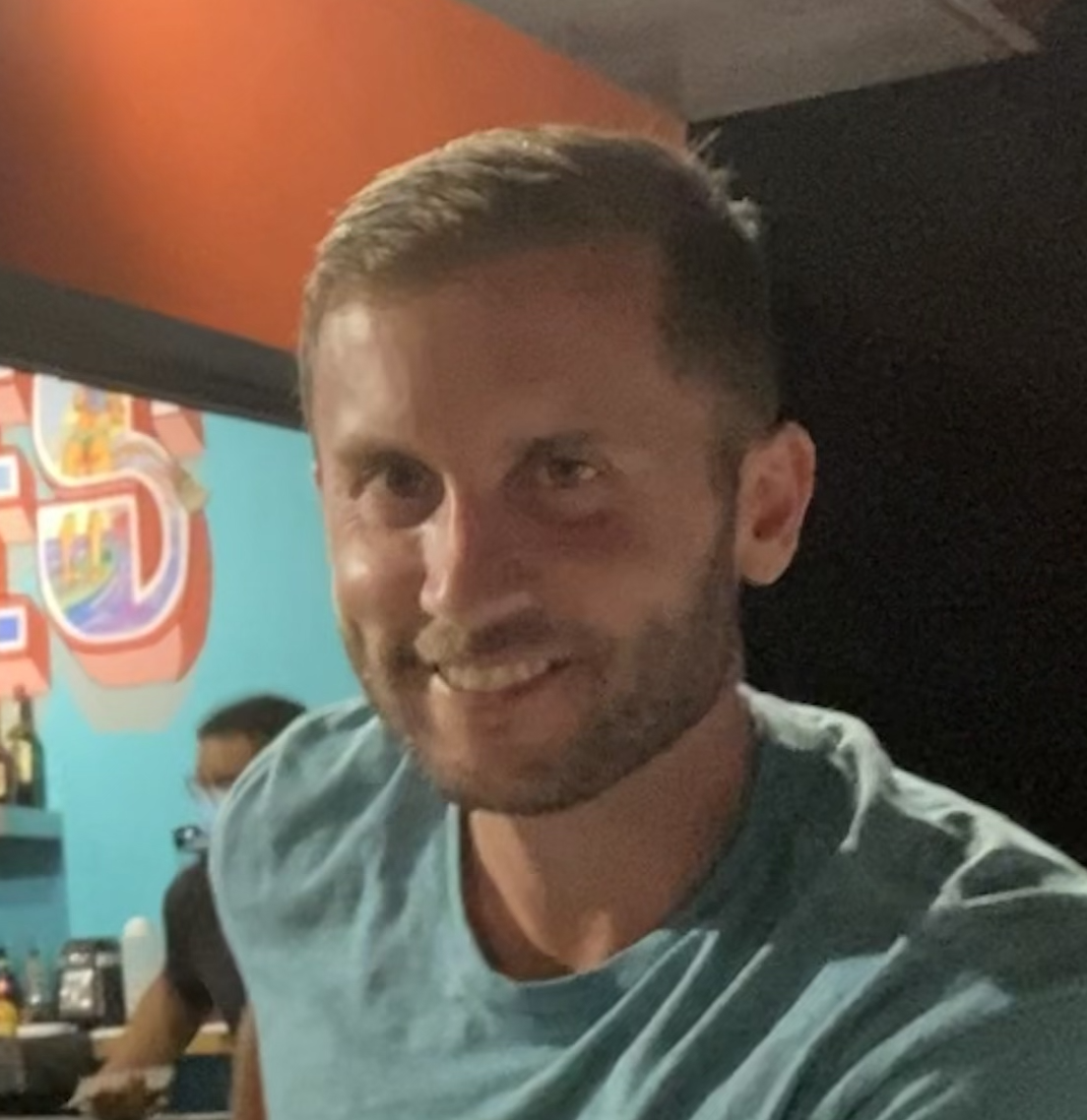
John is a professional software engineer who has been solving problems with code for 15+ years. He has experience with full stack web development, container orchestration, mobile development, DevOps, Windows and Linux kernel development, cybersecurity, and reverse engineering. In his spare time, he’s researching the potential business applications of AI.