This error means you are trying to access a property of a variable that is undefined. To fix this, you should make sure the variable is defined and has that property before you try to access it. You can accomplish this with the question mark operator or an if statement.
Table of Contents
Problem: you are trying to access a field on a variable that is undefined
Let’s say you have the below variable foo
and you set it to undefined
, then try to access the property bar
on it:
const foo = undefined;
console.log(foo.bar);
JavaScriptThis will result in the following error:
/home/user/index.js:3
console.log(foo.bar);
^
TypeError: Cannot read properties of undefined (reading 'bar')
at Object.<anonymous> (/home/user/index.js:3:17)
at Module._compile (node:internal/modules/cjs/loader:1275:14)
at Module._extensions..js (node:internal/modules/cjs/loader:1329:10)
at Module.load (node:internal/modules/cjs/loader:1133:32)
at Module._load (node:internal/modules/cjs/loader:972:12)
at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:83:12)
at node:internal/main/run_main_module:23:47
Node.js v19.8.1
Why does this happen? Because there is no foo
and therefore there’s no way to access some property of foo
when it doesn’t exist.
Solution 1: Use the question mark operator
We can fix this by using the new-ish “question mark” operator. I’m not sure if that’s the scientific name for it, but that’s what I call it.
Here’s how you do that:
const foo = undefined;
console.log(foo?.bar);
// -> undefined
JavaScriptSo what’s going on here? What we’re doing is saying “if foo
exists, then access .bar
“, but all in one line. That’s all there is to it. If foo
doesn’t exist, the expression foo?.bar
will resolve to undefined
, thus preventing a stack trace.
Solution 2: Use an if statement to check for undefined first
Another way we can do this is with an if
statement. This is very straightforward to implement. Here’s how you would do that:
const foo = undefined;
if (foo !== undefined) {
console.log(foo.bar);
}
JavaScriptOne thing to note here, is that we are sure foo exists before trying to access the property bar
on it, but we aren’t sure that bar
is a property of foo
. So, depending on your application, that might be worth checking on as well before you try to access it.
Conclusion
The Javascript error “TypeError: Cannot read properties of undefined” just means you’re trying to access a property on a variable that isn’t defined. The way to fix this is to check for that state first, and to not attempt accessing it if it’s not defined.
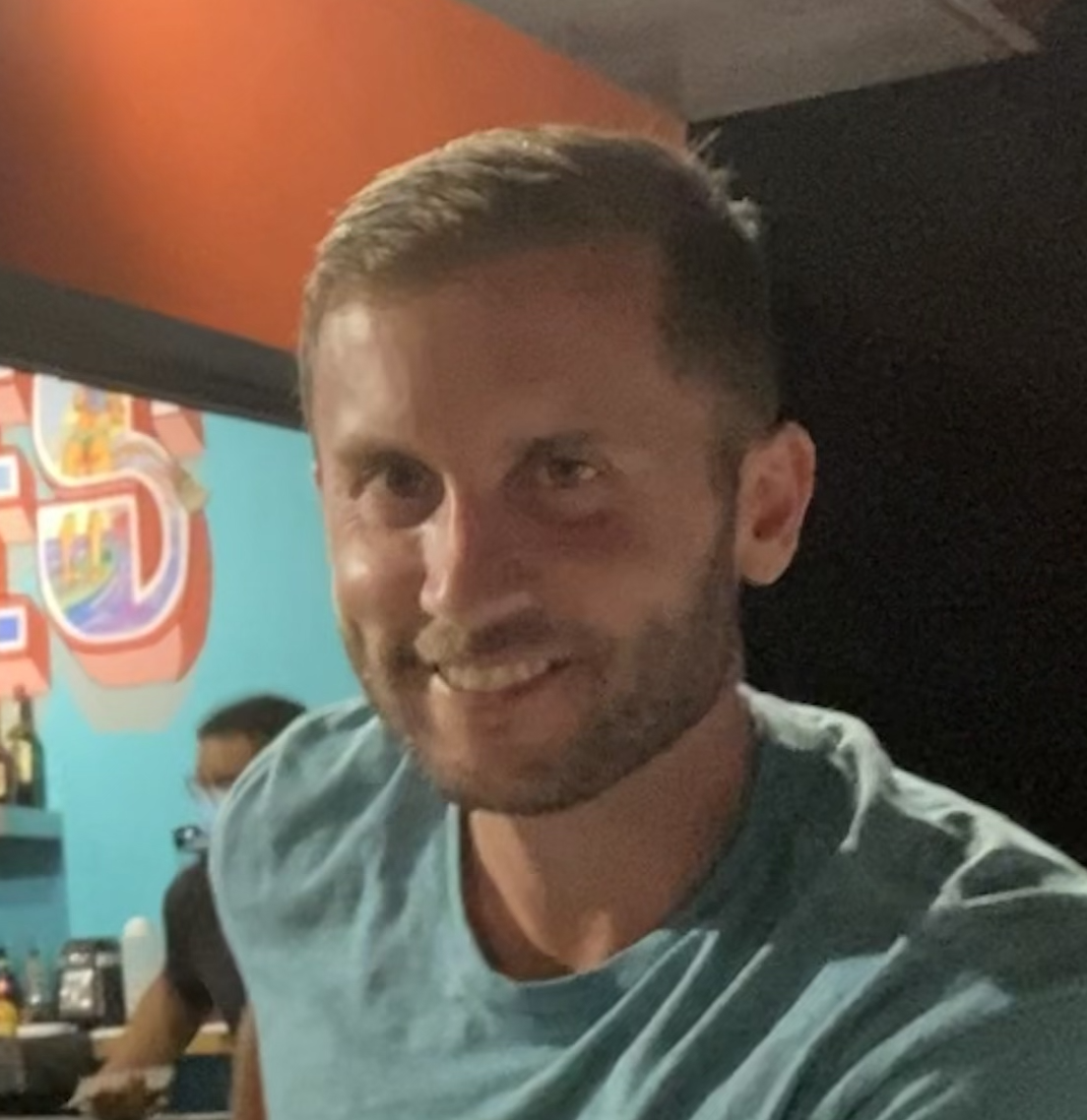
John is a professional software engineer who has been solving problems with code for 15+ years. He has experience with full stack web development, container orchestration, mobile development, DevOps, Windows and Linux kernel development, cybersecurity, and reverse engineering. In his spare time, he’s researching the potential business applications of AI.